1 Material database for power electronic usage
The main purpose of the material database is to provide various materials for FEM simulations or other calculations in which material data from data sheets or own measurements are required.
Possible application scenarios:
FEM Magnetics Toolbox (FEMMT) loads the permeability or the conductivity of the core from the database, depending on the material.
Graphical user interface (GUI) in FEMMT can compare properties of the material stored in material database.
1.1 Overview features
1.1.1 Usable features
Human-readable database based on a .json-file
- Input features:
- Write magnetic parameters into the database
Amplitude of permeability
Angle of permeability
Power loss density (hysteresis losses)
Magnetic flux density
Magnetic field strength
- Write electric parameters into the database
Amplitude of permittivity
Angle of permittivity
Power loss density (eddy current losses)
Electric flux density
Electric field strength
Write datasheet data into the database
- Output features:
Get the magnetic parameters from the database
Providing permeability and permittivity data for FEMMT
Interpolation of material data (both electric and magnetic parameters)
- GUI features (included in FEMMT):
Compare the datasheet values of different ferrite cores (e.g. BH-curves or power-loss curves)
- Materials for comparison:
N95
N87
N49
PC200
DMR96A
1.1.2 Planned features (Roadmap for 202x)
- Input features:
Universal function to write data into the database
- Output features:
Get the electric parameters from the database
Extract data from the database as a specific data file (e.g. .csv)
- Plotting features:
Plot the data of a specific ferrite material, e.g. the amplitude of the permeability over the magnetic flux density
- Filter features:
Get all available data for specific filter keys (e.g. temperature, frequency, material etc.)
Filter for some specific value intervals (e.g. 10mT < B-flux < 30mT)
1.2 Installation
pip install materialdatabase
1.3 Basic usage and minimal example
Material properties:
material_db = mdb.MaterialDatabase()
materials = material_db.material_list_in_database()
initial_u_r_abs = material_db.get_material_property(material_name="N95", property="initial_permeability")
core_material_resistivity = material_db.get_material_property(material_name="N95", property="resistivity")
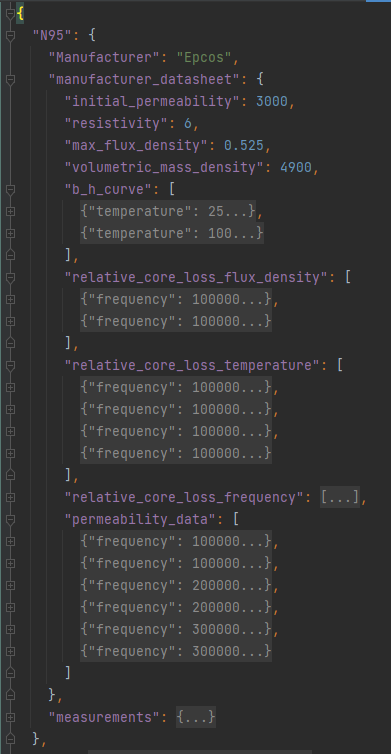
Interpolated permeability and permittivity data of a Material:
b_ref, mu_r_real, mu_r_imag = material_db.permeability_data_to_pro_file(temperature=25, frequency=150000, material_name = "N95", datatype = "complex_permeability",
datasource = mdb.MaterialDataSource.ManufacturerDatasheet, parent_directory = "")
epsilon_r, epsilon_phi_deg = material_db.get_permittivity(temperature= 25, frequency=150000, material_name = "N95", datasource = "measurements",
datatype = mdb.MeasurementDataType.ComplexPermittivity, measurement_setup = "LEA_LK",interpolation_type = "linear")
These function return complex permittivity and permeability for a certain operation point defined by temperature and frequency.
1.4 GUI (FEMMT)
The materials in database can be compared with help GUI in FEM magnetics toolbox. In database tab of GUI, the loss graphs and B-H curves from the datasheets of up to 5 materials can be compared.
FEMMT can be installed using the python pip package manager.
pip install femmt
For working with the latest version, refer to the documentation
1.5 Bug Reports
Please use the issues report button within github to report bugs.
1.6 Changelog
Find the changelog here.
2 Materialdatabase function documentation
Functions of the material database.
- materialdatabase.material_data_base_functions.calc_electric_field_strength_from_lecroy_voltage_data(voltage: ndarray | list, height: float = 1.0)
Calculate the electric field strength based on the voltage data of a lecroy oscilloscope.
- Parameters:
voltage (list or ndarray) – array-like of the voltage in V
height (float) – height of probe in m
- Returns:
array of electric field strength values
- materialdatabase.material_data_base_functions.calc_electric_flux_density_based_on_current_array_and_frequency(current: ndarray | list, frequency: float = 1.0, cross_section: float = 1.0)
Calculate the electric flux density based on the current and the frequency.
- Parameters:
current (ndarray or list) – array-like of the current in A
frequency (float) – frequency value in Hz
cross_section (float) – cross-section of probe in m^2
- Returns:
arrray of electric flux density values
- materialdatabase.material_data_base_functions.calc_electric_flux_density_based_on_current_array_and_time_array(current_array: ndarray | list, time: ndarray | list, cross_section: float = 1.0)
Calculate the electric flux density based on the current data of a lecroy oscilloscope.
- Parameters:
current_array (ndarray or list) – array-like of the current in A
time (ndarray or list) – array of time values of measurement in s
cross_section (float) – cross-section of probe in m^2
- Returns:
array of electric flux density values
- materialdatabase.material_data_base_functions.calc_magnetic_field_strength_based_on_current_array(current: ndarray | list, primary_winding: int = 1, l_mag: float = 1.0)
Calculate the magnetic field strength based on the current.
- Parameters:
current (ndarray or list) – array-like of the current in A
primary_winding (int) – number of primary windings
l_mag (float) – mean magnetic path length in m
- Returns:
array with magnetic field strength curve in A/m
- materialdatabase.material_data_base_functions.calc_magnetic_flux_density_based_on_voltage_array_and_frequency(voltage: ndarray | list, frequency: float = 1.0, secondary_winding: int = 1, cross_section: float = 1.0)
Calculate the magnetic flux density based on the voltage and the frequency.
ASSUMPTION: EXACTLY ONE PERIOD! Based on the length of the voltage array and the frequency the time-array is constructed for the integration.
- Parameters:
voltage (ndarray or list) – array-like of the voltage in V
frequency (float) – frequency value in Hz
secondary_winding (int) – number of secondary windings
cross_section (float) – value of the cross-section of the core in m^2
- Returns:
array with magnetic flux density curve in T
- materialdatabase.material_data_base_functions.calc_magnetic_flux_density_based_on_voltage_array_and_time_array(voltage: ndarray | list, time: ndarray | list, secondary_winding: int = 1, cross_section: float = 1)
Calculate the magnetic flux density based on the voltage and the time.
ASSUMPTION: EXACTLY ONE PERIOD!
- Parameters:
voltage (ndarray or list) – array-like of the voltage in V
time (ndarray or list) – array-like of the time in s
secondary_winding (int) – number of secondary windings
cross_section (float) – value of the cross-section of the core in m^2
- Returns:
array with magnetic flux density curve in T
- materialdatabase.material_data_base_functions.calc_mu_r_from_b_and_h_array(b: ndarray | list, h: ndarray | list)
Calculate the amplitude of the relative permeability based on a magnetic flux density and a magnetic field strength array.
- Parameters:
b (ndarray or list) – magnetic flux density array
h (ndarray or list) – magnetic field strength array
- Returns:
amplitude of the relative permability
- materialdatabase.material_data_base_functions.check_input_permeability_data(datasource: str, material_name: str, temperature: float, frequency: float) None
Check input permeability data for correct input parameters.
datasource must be ‘measurements’ or ‘manufacturer_datasheet’
material_name, T, f must be different from None
- Parameters:
datasource (str) – datasource as a string
material_name (str) – material name as a string
temperature (float) – temperature in °C
frequency (float) – frequency in Hz
- materialdatabase.material_data_base_functions.clear_permeability_measurement_data_in_database(material_name: str, measurement_setup: str)
Clear the permeability data in the database given a material and measurement setup.
- Parameters:
material_name (str) – name of the material
measurement_setup (str) – name of the measurement setup
- materialdatabase.material_data_base_functions.clear_permittivity_measurement_data_in_database(material_name: str, measurement_setup: str)
Clear the permittivity data in the database for a specific material.
- Parameters:
material_name (str) – name of material
measurement_setup (str) – name of measurement setup
- materialdatabase.material_data_base_functions.create_empty_material(material_name: str, manufacturer: str, initial_permeability: float, resistivity: float, max_flux_density: float, volumetric_mass_density: float)
Create an empty material slot in the database.
- Parameters:
material_name (str) – name of the material
manufacturer (str) – name of the manufacturer
initial_permeability (float) – value of the initial permeability
resistivity (float) – value of the resistivity
max_flux_density (float) – saturation value of the magnetic flux density
volumetric_mass_density (float) – value of the volumetric mass density
- materialdatabase.material_data_base_functions.create_permeability_file_name_lea_lk(quantity: str = 'p_hys', frequency: float = 100000, material_name: str = 'N49', temperature: float = 30)
Create the file name for permeability data of LEA_LK.
- Parameters:
quantity (str) – measured quantiy (e.g. p_hys)
material_name (str) – name of the material
frequency (float) – frequency value in Hz
temperature (float) – temperature value in °C
- Returns:
correct file name for LEA_LK
- materialdatabase.material_data_base_functions.create_permeability_measurement_in_database(material_name: str, measurement_setup: str, company: str = '', date: str = '', test_setup_name: str = '', toroid_dimensions: str = '', measurement_method: str = '', equipment_names: str = '', comment: str = '')
Create a new permeability section in the database for a material.
- Parameters:
material_name (str) – name of the material
measurement_setup (str) – name of the measurement setup
company (str) – name of the company
date (str) – date of measurement
test_setup_name (str) – information of the test setup
toroid_dimensions (str) – dimensions of the probe
measurement_method (str) – name of the measurement method
equipment_names (str) – name of the measurement equipment
comment (str) – comment regarding the measurement
- materialdatabase.material_data_base_functions.create_permeability_neighbourhood_datasheet(temperature: float, frequency: float, list_of_permeability_dicts: ndarray | list)
Create a neighbourhood for permeability data of a datasheet.
- Parameters:
temperature (float) – temperature value in °C
frequency (float) – frequency value in Hz
list_of_permeability_dicts (ndarray or list) – list of permeability data dicts
- Returns:
neighbourhood
- materialdatabase.material_data_base_functions.create_permeability_neighbourhood_measurement(temperature: float, frequency: float, list_of_permeability_dicts: ndarray | list)
Create a neighbourhood for permeability data of a measurement.
- Parameters:
temperature (float) – temperature value in °C
frequency (float) – frequency value in Hz
list_of_permeability_dicts (ndarray or list) – list of permeability dicts
- Returns:
neighbourhood
- materialdatabase.material_data_base_functions.create_permittivity_file_name_lea_lk(quantity: str = 'p_hys', frequency: float = 100000, material_name: str = 'N49', temperature: float = 30)
Create the file name for permittivity data of LEA_LK.
- Parameters:
quantity (str) – measured quantiy (e.g. p_hys)
frequency (float) – frequency value in Hz
material_name (str) – name of the material
temperature (float) – temperature value in °C
- Returns:
correct file name for LEA_LK
- materialdatabase.material_data_base_functions.create_permittivity_measurement_in_database(material_name: str, measurement_setup: str, company: str = '', date: str = '', test_setup_name: str = '', probe_dimensions: str = '', measurement_method: str = '', equipment_names: str = '', comment: str = '')
Create a new permittvity section in the database for a material.
- Parameters:
material_name (str) – name of the material
measurement_setup – name of the measurement setup
company (str) – name of the company
date (str) – date of measurement
test_setup_name (str) – information of the test setup
probe_dimensions (str) – dimensions of the probe
measurement_method (str) – name of the measurement method
equipment_names (str) – name of the measurement equipment
comment (str) – comment regarding the measurement
- materialdatabase.material_data_base_functions.create_permittivity_neighbourhood(temperature: float, frequency: float, list_of_permittivity_dicts: ndarray | list)
Create neighbourhood for permittivity data.
- Parameters:
temperature (float) – temperature value in °C
frequency (float) – frequency value in Hz
list_of_permittivity_dicts (ndarray or list) – list of permittivity data dicts
- Returns:
neighbourhood
- materialdatabase.material_data_base_functions.create_steinmetz_neighbourhood(temperature: float, list_of_steinmetz_dicts: ndarray | list)
Create neighbourhood for steinmetz data.
- Parameters:
temperature (float) – temperature value in °C
list_of_steinmetz_dicts (ndarray or list) – list of steinmetz data dicts
- Returns:
neighbourhood
- materialdatabase.material_data_base_functions.crop_3_with_1(x: ndarray | list, y: ndarray | list, z: ndarray | list, xa: int | float, xb: int | float)
Crop three arrays based on one array.
- Parameters:
x (ndarray or list) – array crop is based on
y (ndarray or list) – first array to get cropped
z (ndarray or list) – second array to get cropped
xa (float or int) – start value of crop
xb (float or int) – end value of crop
- Returns:
the three cropped arrays
- materialdatabase.material_data_base_functions.crop_data_fixed(x: list, pre_cropped_values: int = 0, post_cropped_values: int = 0)
Crop an array based on the given indices.
IMPORTANT! THE SECOND INDEX IS COUNTED BACKWARDS(NEGATIVE)!
- Parameters:
x (list) – array to get cropped
pre_cropped_values (int) – start value
post_cropped_values (int) – end value, but counted backwards
- Returns:
cropped data
- materialdatabase.material_data_base_functions.eps_phi_deg__from_eps_r_and_p_eddy(frequency: ndarray | float, e_peak: ndarray | float, eps_r: ndarray | float, p_eddy: ndarray | float)
Calculate the angle of the permittivity.
- Parameters:
frequency (ndarray or float) – frequency
e_peak (ndarray or float) – peak value of the electric field strength
eps_r (ndarray or float) – peak value of the amplitude of the permittivity
p_eddy (ndarray or float) – eddy current loss density
- Returns:
angle of permittivity in degree
- materialdatabase.material_data_base_functions.export_data(parent_directory: str = '', file_format: str | None = None, b_ref_vec: ndarray | list | None = None, mu_r_real_vec: ndarray | list | None = None, mu_r_imag_vec: ndarray | list | None = None, silent: bool = False)
Export data from the material database in a certain file format.
- Parameters:
parent_directory (str) – path to parent directory
file_format (str) – export format, e.g. ‘pro’ to export a .pro-file
b_ref_vec (ndarray or list) – reference vector for mu_r_real and mu_r_imag
mu_r_real_vec (ndarray or list) – real part of mu_r_abs as a vector
mu_r_imag_vec (ndarray or list) – imaginary part of mu_r_abs as a vector
silent (bool) – enables/disables print
- materialdatabase.material_data_base_functions.find_nearest(array: ndarray | list, value: float)
Find the nearest value in an array.
- Parameters:
array (ndarray or list) – array to search
value (float) – desired value
- Returns:
two values of the array with the wanted value in between
- materialdatabase.material_data_base_functions.find_nearest_frequencies(permeability: ndarray | list, frequency: float)
Find the nearest frequency value for permeability data.
- Parameters:
permeability (ndarray or list) – permeability data
frequency (float) – desired frequency value in Hz
- Returns:
two frequency values in Hz with the desired value in between
- materialdatabase.material_data_base_functions.find_nearest_neighbour_values_permeability(permeability_data: ndarray | list, temperature: float, frequency: float)
Find the nearest temperature and frequency values for a given neighbourhood of permeability data.
- Parameters:
permeability_data (ndarray or list) – permeability data
temperature (float) – temperature value in °C
frequency (float) – frequency value in Hz
- Returns:
lower temperature value in °C, higher temperature value in °C, lower frequency value in Hz, higher frequency value in Hz
- materialdatabase.material_data_base_functions.find_nearest_neighbours(value: float, list_to_search_in: ndarray | list)
Return the two values with the wanted value in between and additional the indices of the corresponding values.
Only works for sorted lists (small to big).
Case 0: if len(list_to_search_in) == 1: return duplicated Case 1: if value == any(list_to_search_in): return duplicated Case 2: if value inbetween: return neighbours Case 3a: value smaller than data: return smallest two Case 3b: if value is bigger than data: return biggest two
- Parameters:
value (float) – desired value
list_to_search_in (ndarray or list) – array to search for value
- Returns:
lower index, lower value, higher index, higher value
- materialdatabase.material_data_base_functions.find_nearest_temperatures(permeability: ndarray | list, f_l: float, f_h: float, temperature: float)
Find the nearest temperature value between two frequency points.
- Parameters:
permeability (ndarray or list) – permeability data
f_l (float) – lower frequency value in Hz
f_h (float) – higher frequency value in Hz
temperature (float) – desired temperature value in °C
- Returns:
two temperature values in °C with the desired value in between
- materialdatabase.material_data_base_functions.get_all_frequencies_for_material(material_path: str)
Get all the frequency values for a given material.
- Parameters:
material_path (str) – path to the material
- Returns:
all frequency values in Hz of the given material
- materialdatabase.material_data_base_functions.get_all_temperatures_for_directory(toroid_path: str)
Get all the temperature values for a given toroid probe.
- Parameters:
toroid_path (str) – path of the toroid probe
- Returns:
all temperature values in °C of the specific toroid probe
- materialdatabase.material_data_base_functions.get_bh_integral_shoelace(b: ndarray, h: ndarray, f: float)
Calculate the hysteresis loss density.
- Parameters:
b (ndarray) – magnetic flux density in T
h (ndarray) – magnetic field strength in A/m
f (float) – frequency in Hz
- Returns:
hysteresis loss density in W/m^3
- materialdatabase.material_data_base_functions.get_bh_integral_trapezoid(b: ndarray, h: ndarray, f: float)
Calculate the hysteresis loss density.
- Parameters:
b (ndarray) – magnetic flux density in T
h (ndarray) – magnetic field strength in A/m
f (float) – frequency in Hz
- Returns:
hysteresis loss density in W/m^3
- materialdatabase.material_data_base_functions.get_permeability_data_from_lea_lk(location: str, frequency: float, temperature: float, material_name: str, no_interpolation_values: int = 20)
Get the permeability data from LEA_LK.
- Parameters:
location (str) – location of the permeability data
frequency (float) – frequency value in Hz
temperature (float) – temperature value in °C
material_name (str) – name of the material
no_interpolation_values (int) – number of interpolation values
- Returns:
magnetic flux density, amplitude of the permeability, angle of the permeability
- materialdatabase.material_data_base_functions.get_permeability_data_from_lea_mtb(location: str)
Get the permeability data from the material test bench.
- Parameters:
location (str) – location of the permability data
- Returns:
magnetic flux density, amplitude of the permeability, angle of the permeability
- materialdatabase.material_data_base_functions.get_permeability_property_from_lea_lk(path_to_parent_folder: str, quantity: str, frequency: float, material_name: str, temperature: float, sub_folder_name: str = 'Core_Loss')
Get the proberty of the permeability from LEA_LK.
- Parameters:
path_to_parent_folder (str) – path to permeability data
quantity (str) – name of the measured quantity
material_name (str) – name of the material
frequency (float) – frequency value in Hz
temperature (float) – temperature value in °C
sub_folder_name (str) – name of the sub folder
- Returns:
amplitude of the permeability, angle of the permeability
- materialdatabase.material_data_base_functions.get_permeability_property_from_lea_mtb(path_to_parent_folder: str)
Get the proberty of the permeability from the material test bench.
- Parameters:
path_to_parent_folder (str) – path to permeability data
- Returns:
magnetic flux density, amplitude of the permeability, angle of the permeability
- materialdatabase.material_data_base_functions.get_permittivity_data_from_lea_lk(location: str, temperature: float, frequency: float, material_name: str)
Get the permittivity data from LEA_LK.
- Parameters:
location (str) – location of the permittivity data
temperature (float) – temperature value
frequency (float) – frequency value in Hz
material_name (str) – name of the material
- Returns:
amplitude of the permittivity, angle of the permittivity
- materialdatabase.material_data_base_functions.get_permittivity_property_from_lea_lk(path_to_parent_folder: str, quantity: str, frequency: float, material_name: str, temperature: float, sub_folder_name: str = 'Core_Loss')
Get the proberty of the permittivity from LEA_LK.
- Parameters:
path_to_parent_folder (str) – path to permittivity data:
quantity (str) – name of the measured quantity
frequency (float) – frequency value in Hz
material_name (str) – name of the material
temperature (float) – temperature value in °C
sub_folder_name (str) – name of the sub folder
- Returns:
amplitude of the permittivity, angle of the permittivity
- materialdatabase.material_data_base_functions.getdata_datasheet(permeability: ndarray | list, variable: float, frequency: float, temperature_1: float, temperature_2: float)
Interpolation of permeability data between two temperatures at a constant frequency.
Linear Interpolation between temperature_1 and temperature_2 to get a value for the temperature “variable”.
- Parameters:
permeability (ndarray or list) – permeability data
variable (float) – desired temperature value in °C
frequency (float) – frequency value in Hz
temperature_1 (float) – first temperature value in °C
temperature_2 (float) – second temperature value in °C
- Returns:
magnetic flux density, real part of permeability and imaginary part of permeability
- materialdatabase.material_data_base_functions.getdata_measurements(permeability: ndarray | list, variable: float, frequency: float, temperature_1: float, temperature_2: float, b_t: ndarray | list)
Linear interpolation of the permeability data between two temperatures at a constant frequency.
- Parameters:
permeability (ndarray or list) – permeability data
variable (float) – desired temperature variable in °C
frequency (float) – frequency value in Hz
temperature_1 (float) – temperature value under the desired value in °C
temperature_2 (float) – temperature value above the desired value in °C
b_t (ndarray or list) – magnetic flux density
- Returns:
amplitude of the permeability, angle of the permeability
- materialdatabase.material_data_base_functions.integrate(x_data: ndarray | list, y_data: ndarray | list)
Integrate the function y_data = f(x_data).
- Parameters:
x_data (ndarray or list) – x-axis
y_data (ndarray or list) – y-axis
- Returns:
defined integral of y_data
- materialdatabase.material_data_base_functions.interpolate_a_b_c(a: ndarray | list, b: ndarray | list, c: ndarray | list, no_interpolation_values: int = 20)
Interpolation between three arrays based on the first array.
- Parameters:
a (ndarray or list) – array that is the base of the interpolation
b (ndarray or list) – array that gets interpolated based on the values of array a
c (ndarray or list) – array that gets interpolated based on the values of array a
no_interpolation_values (int) – number of interpolation values
- Returns:
the three interpolated arrays
- materialdatabase.material_data_base_functions.interpolate_b_dependent_quantity_in_temperature_and_frequency(temperature: float, frequency: float, temperature_low: float, temperature_high: float, frequency_low: float, frequency_high: float, b_t_low_f_low: ndarray, f_b_T_low_f_low: ndarray, b_T_high_f_low: ndarray, f_b_T_high_f_low: ndarray, b_T_low_f_high: ndarray, f_b_T_low_f_high: ndarray, b_T_high_f_high: ndarray, f_b_T_high_f_high: ndarray, no_interpolation_values: int = 8, y_label: str | None = None, plot: bool = False)
Interpolate a magnet flux density dependent quantity in temperature and frequency.
- Parameters:
temperature (float) – desired temperature in °C
frequency (float) – desired frequency in Hz
temperature_low (float) – lower temperature value in °C
temperature_high (float) – higher temperature value in °C
frequency_low (float) – lower frequency value in Hz
frequency_high (float) – higher frequency value in Hz
b_t_low_f_low (ndarray) – magnetic flux density at the lower temperature in °C and the lower frequency value in Hz
f_b_T_low_f_low (ndarray) – function dependent of b at the lower temperature in °C and the lower frequency value in Hz
b_T_high_f_low (ndarray) – magnetic flux density at the higher temperature in °C and the lower frequency value in Hz
f_b_T_high_f_low (ndarray) – function dependent of b at the higher temperature in °C and the lower frequency value in Hz
b_T_low_f_high (ndarray) – magnetic flux density at the lower temperature in °C and the higher frequency value in Hz
f_b_T_low_f_high (ndarray) – function dependent of b at the lower temperature in °C and the higher frequency value in Hz
b_T_high_f_high (ndarray) – magnetic flux density at the higher temperature in °C and the higher frequency value in Hz
f_b_T_high_f_high (ndarray) – function dependent of b at the higher temperature in °C and the higher frequency value in Hz
no_interpolation_values (int) – number of interpolation values
y_label (str) – label of y-axes
plot (bool) – enable/disable plotting of data
- Returns:
array of magnetic flux density, arrays of function dependent of b
- materialdatabase.material_data_base_functions.interpolate_neighbours_linear(temperature: float, frequency: float, neighbours: dict)
Linear interpolation of frequency and temperature between neighbours.
- Parameters:
temperature (float) – desired temperature value in °C
frequency (float) – desired frequency value in Hz
neighbours (dict) – neighbours
- Returns:
amplitude of the permittivity, angle of the permittivity in degree
- materialdatabase.material_data_base_functions.load_material_from_db(material_name: str) None
Load data from material database.
- Parameters:
material_name (str) – name of material
- Returns:
all data of specific material
- Return type:
dict
- materialdatabase.material_data_base_functions.mu_phi_deg__from_mu_r_and_p_hyst(frequency: ndarray | float, b_peak: ndarray | float, mu_r: ndarray | float, p_hyst: ndarray | float)
Calculate the phase angle of the permeability given the peak value of the magnetic flux density, the hysteresis loss and the amplitude of permeability.
- Parameters:
frequency (ndarray or float) – frequency in Hz
b_peak (ndarray or float) – peak flux density in T
mu_r (ndarray or float) – amplitude of the permeability in unitless
p_hyst (ndarray or float) – hysteresis losses in W/m^3
- Returns:
phase angle of the permeability in degree
- materialdatabase.material_data_base_functions.mu_r__from_p_hyst_and_mu_phi_deg(mu_phi_deg: ndarray | float, frequency: ndarray | float, b_peak: ndarray | float, p_hyst: ndarray | float)
Calculate the amplitude of the permeability given the peak value of the magnetic flux density, the hysteresis loss and the phase angle of the permeability.
- Parameters:
mu_phi_deg (ndarray or float) – phase angle of the permeability in degree
frequency (ndarray or float) – frequency in Hz
b_peak (ndarray or float) – peak flux density in T
p_hyst (ndarray or float) – hysteresis losses in W/m^3
- Returns:
amplitude of the permeability
- materialdatabase.material_data_base_functions.my_interpolate_linear(a: float, b: float, f_a: float, f_b: float, x: float)
Interpolates linear between to points ‘a’ and ‘b’.
The return value is f_x in dependence of x It applies: a < x < b.
- Parameters:
a (float) – x-value for point a
b (float) – x-value for point b
f_a (float) – y-value for point a
f_b (float) – y-value for point b
x (float) – x-value for the searched answer f_x
- Returns:
y-value for given x-value
- materialdatabase.material_data_base_functions.my_polate_linear(a: float, b: float, f_a: float, f_b: float, x: float)
Interpolates or extrapolates linear for a<x<b or x<a and x>b.
- Parameters:
a (float) – input x-value for point a
b (float) – input x-value for point b
f_a (float) – input y-value for point a
f_b (float) – input y-value for point b
x (float) – x-value for the searched answer f_x
- Returns:
y-value for given x-value
- materialdatabase.material_data_base_functions.p_hyst__from_mu_r_and_mu_phi_deg(frequency: ndarray | float, b_peak: ndarray | float, mu_r: ndarray | float, mu_phi_deg: ndarray | float)
Calculate the hysteresis losses given the peak value of the magnetic flux density, the amplitude and phase angle of the permeability.
- Parameters:
frequency (ndarray or float) – frequency in Hz
b_peak (ndarray or float) – peak flux density in T
mu_r (ndarray or float) – amplitude of the permeability in unitless
mu_phi_deg (ndarray or float) – phase angle of the permeability in degree
- Returns:
hysteresis losses in W/m^3
- materialdatabase.material_data_base_functions.plot_data(material_name: str | None = None, properties: str | None = None, b_ref: ndarray | list | None = None, mu_r_real: ndarray | list | None = None, mu_r_imag: ndarray | list | None = None)
Plot certain material properties of materials.
TODO: parameter is new and will probably cause problems when plotting data, but previous implementation was very static… :param material_name: name of the material :type material_name: str :param properties: name of the material properties :type properties: str :param b_ref: magnetic flux density value: :type b_ref: ndarray or list :param mu_r_real: real part of the permeability :type mu_r_real: ndarray or list :param mu_r_imag: imaginary part of the permeability :type mu_r_imag: ndarray or list
- materialdatabase.material_data_base_functions.process_permeability_data(b_ref_raw: ndarray | list, mu_r_raw: ndarray | list, mu_phi_deg_raw: ndarray | list, b_min: float = 0.05, b_max: float = 0.3, smooth_data: bool = False, crop_data: bool = False, plot_data: bool = False, ax=None, f: float | None = None, T: float | None = None)
Post-Processing of raw data of the permeability.
Function can smooth, crop and plot the permeability data.
- Parameters:
b_ref_raw (ndarray or float) – raw data of the magnetic flux density
mu_r_raw (ndarray or float) – raw data of the amplitude of the permeability
mu_phi_deg_raw (ndarray or float) – raw data of the angle of the permeability
b_min (float) – min value of the magnetic flux density for cropping
b_max (float) – max value of the magnetic flux density for cropping
smooth_data (bool) – enable/disable smoothing of data (savgol-filter)
crop_data (bool) – enable/disable cropping of data
plot_data (bool) – enable/disable plotting of data
ax (matplotlib.axes) – axes for plot
f (float) – frequency value in Hz
T (float) – temperature value in °C
- Returns:
magnetic flux density and amplitude and angle of permeability
- materialdatabase.material_data_base_functions.read_in_digitized_datasheet_plot(path: str)
Read in a csv-file containing the x- and y-data of a digitized plot from a manufacturer datasheet.
- Information regarding Digitization:
used program: WebPlotDigitizer (made by Ankit Rohatgi)
delta_x = 8 Px
delta_y = 8 Px
- format to save: Sort by: X
Order: Ascending Digits: 5 Fixed Column Separator: ;
- Parameters:
path (str) – path to csv-file
- Returns:
list containing two lists with x- and y-data ([[“x-data”], [“y-data”]])
- materialdatabase.material_data_base_functions.rect(radius_or_amplitude: ndarray | float, theta_deg: ndarray | float)
Convert polar coordinates [radius, angle] into cartesian coordinates [abscissa_x,ordinate_y].
- Parameters:
radius_or_amplitude (ndarray or float) – radius or amplitude
theta_deg (ndarray or float) – angle in degree
- Returns:
abscissa_x, ordinate_y
- materialdatabase.material_data_base_functions.remove(arr: ndarray, n: int)
Remove duplicates from array.
- Parameters:
arr (ndarray) – array with duplicates
n (int) – has no effect of the functionality
- Returns:
array without duplicates
- materialdatabase.material_data_base_functions.remove_mean_of_signal(signal: ndarray | None = None)
Remove the mean value of a signal.
- Parameters:
signal (ndarray) – signal with mean value
- Returns:
signal without mean value
- materialdatabase.material_data_base_functions.sigma_from_permittivity(amplitude_relative_equivalent_permittivity: ndarray | float, phi_deg_relative_equivalent_permittivity: ndarray | float, frequency: ndarray | float)
Calculate the conductivity based on the data of the permittivity.
- Parameters:
amplitude_relative_equivalent_permittivity (ndarray or float) – amplitude of the permittivity
phi_deg_relative_equivalent_permittivity (ndarray or float) – angle of the permittivity
frequency (nd array or float) – frequency value in Hz
- Returns:
conductivity
- materialdatabase.material_data_base_functions.sort_data(a: ndarray | list, b: ndarray | list, c: ndarray | list)
Sort three arrays according to array a.
- Parameters:
a (ndarray or list) – array that is the base of the sorting
b (ndarray or list) – array that gets sorted based on a
c (ndarray or list) – array that gets sorted based on a
- Returns:
the three arrays sorted according to a
- materialdatabase.material_data_base_functions.store_data(material_name: str, data_to_be_stored: dict) None
Store data from measurement/datasheet into the material database.
- Parameters:
material_name (str) – name of the material
data_to_be_stored (dict) – data to be stored
- materialdatabase.material_data_base_functions.updates_x_ticks_for_graph(x_data: ndarray | list, y_data: ndarray | list, x_new: ndarray | list | float, kind: str = 'linear')
Update the x-values of the given (x_data,y_data)-dataset and returns y_new based on x_new.
- Parameters:
x_data (ndarray or list) – x-data given
y_data (ndarray or list) – y-data given
x_new (ndarray or list or float) – new x-values
kind (str) – kind of interpolation
- Returns:
y_new-data corresponding to the x_new-data
- materialdatabase.material_data_base_functions.write_permeability_data_into_database(frequency: float, temperature: float, b_ref: ndarray | list, mu_r_abs: ndarray | list, mu_phi_deg: ndarray | list, material_name: str, measurement_setup: str, current_shape: str = 'sine', H_DC_offset: float = 0, overwrite: bool = False)
Write permeability data into the material database.
CAUTION: This method only adds the given measurement series to the permeability data without checking duplicates.
- Parameters:
frequency (float) – frequency value in Hz
temperature (float) – temperature value in °C
b_ref (ndarray or list) – magnetic flux density value
mu_r_abs (ndarray or list) – amplitude of the permeability
mu_phi_deg (ndarray or list) – angle of the permeability
material_name (str) – name of the material
measurement_setup (str) – name of the measurement setup
current_shape (str) – shape of the current (e.g. “sine”, “triangle”, “trapezoid”)
H_DC_offset (float) – offset in the magnetic field strength in A/m
overwrite (bool) – enable/disable overwritting of data
- materialdatabase.material_data_base_functions.write_permittivity_data_into_database(temperature: float, frequencies: ndarray | list, epsilon_r: ndarray | list, epsilon_phi_deg: ndarray | list, material_name: str, measurement_setup: str)
Write permittivity data into the material database.
- Parameters:
temperature (float) – measurement point of the temperature in °C
frequencies (ndarray or list) – measurement points of the frequency in Hz
epsilon_r (ndarray or list) – amplitude of the permittivity
epsilon_phi_deg (ndarray or list) – angle of the permittivity
material_name (str) – name of material
measurement_setup (str) – name of measurement setup
- materialdatabase.material_data_base_functions.write_steinmetz_data_into_database(temperature: float, k: float, beta: float, alpha: float, material_name: str, measurement_setup: str)
Write steinmetz data into the material database.
CAUTION: This method only adds the given measurement series to the steinmetz data without checking duplicates.
- Parameters:
temperature (float) – temperature value in °C
k (float) – k value of steinmetz parameters
beta (float) – beta value of the steinmetz parameters
alpha (float) – alpha value of the steinmetz parameters
material_name (str) – name of the material
measurement_setup (str) – name of the measurement setup