1 Welcome to PyGeckoCircuits2
This repository was inspired by MaurerM/pygeckocircuits, added by certain functions and a pip-installable package.
Control a GeckoCircuits simulation by Python code.
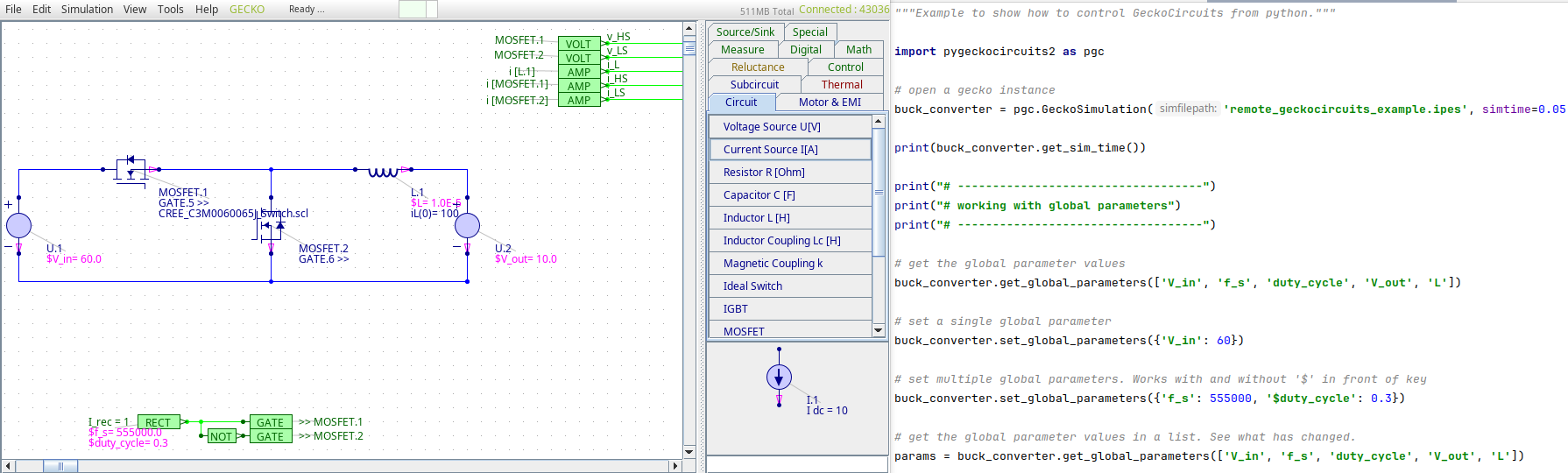
1.1 Installation
Install pygeckocircuits2 directly from pyPI:
pip install pygeckocircuits2
1.2 Usage
Download the compiled gecko-version from tinix84/gecko.
Run the example file to see the given functions.
1.3 Documentation
Find the documentation here.
1.4 Troubleshooting
As of now, there are issues with OpenJDK 21 (LTS). Please use OpenJDK 17 (LTS) so far. See also this issue.
2 PyGeckoCircuits2 class and function documentation
2.1 The GeckoSimulation
class
- class pygeckocircuits2.GeckoSimulation(simfilepath: str, geckoport: int = 43036, timestep: float | None = None, simtime: float | None = None, timestep_pre: float = 0, simtime_pre: float = 0)
A class to control the GeckoCIRCUITS power electronics simulation tool remotely using inbuilt api services via remote connection.
Please ensure that the remote access of GeckoCIRCUITS is enabled and set to 43036 port.
This class is based on the work of https://github.com/MauererM/pygeckocircuits/blob/master/PyGeckoExample.py GeckoCIRCUITS: https://github.com/geckocircuits/GeckoCIRCUITS
- Definitions:
Parameters are global - parameter_key - parameter_value
Every component (e.g. ‘L.1’) contains - a component_key (e.g. ‘L’) - a component_value (e.g. 100e-6)
a node is a net-node in GeckoCIRCUITS, e.g. you can name a net with a label ‘v_in’
- Method naming:
set : e.g. to set values or parameters
get : e.g: to get keys, values or parameters
- Special-members:
__init__
- get_component_keys(component_name: str) List
Return the string list of all component names.
This function is typically not used by the user. Helper-method for get_component_values()
- Parameters:
component_name (str) – the name of the component (ex: IGBT.1)
- Returns:
list of all the accessible component parameter keys
- Return type:
List
- get_component_values(component_name: str) Dict
Return the values of the component parameters.
- Parameters:
component_name (str) – the name of the component (ex: IGBT.1)
- Returns:
values of the component parameters in a dict
- Return type:
Dict
- Example:
>>> import pygeckocircuits2 as pgc >>> gecko_instance = pgc.GeckoSimulation('path/to/simfile.ipes') >>> gecko_instance.get_component_values('mosfet.1')
!NOTE!: all designators and indizees must be chosen with capital letters in the .ipes file
- get_global_parameters(parameters: List | str) Dict
Get the existing value of the provided global parameter variables.
- Parameters:
parameters (List[str] or str) – names of the global parameters (excluding $)
- Returns:
dict with available parameters
- Return type:
Dict
- Example:
>>> import pygeckocircuits2 as pgc >>> buck_converter = pgc.GeckoSimulation('path/to/gecko_file.ipes') >>> params = buck_converter.get_global_parameters(['V_in', 'f_s', 'duty_cycle', 'V_out', 'L'])
- get_scope_data(node_names: List | str, file_name: str, start_time: float | None = None, stop_time: float | None = None, skip_points: int = 0) DataFrame
Get the data from the scope that has been recorded after the corresponding simulation.
The data with respect to specified scope nodes are extracted and save to csv file locally.
- Parameters:
node_names (List[str] or str) – the name provided to the scope nodes, e.g. [“v1”, “i_l_1”]
file_name (str) – name of the csv file under which the extracted data needed to be exported
start_time (float) – the time from where the data needs to be recorded. Defaults to 0 s.
stop_time (float) – the time at which the data recording stops. Defaults to end of simulation time.
skip_points (int) – the length of points that needs to be skipped (ex: skip_points = 2 means data is recorded after every 2 data points)
- Returns:
None
- Return type:
None
- get_sim_time() Tuple
Print and return the current step time and simulation time to the console.
- Returns:
simtime, timestep
- Return type:
Tuple
- get_switch_keys(sw_type: str) List
Configure switch method to set the properties of the selected switch type. This function does _not_ interact with geckoCircuits.
- Parameters:
sw_type (str) – switch type can be either mosfet/igbt/diode
- Returns:
characteristics parameter names as list
- Return type:
List
- Example:
>>> import pygeckocircuits2 as pgc >>> buck_converter = pgc.GeckoSimulation('Example_Gecko.ipes', simtime=0.05, timestep=50e-9) >>> mosfet_parameter_list = buck_converter.get_switch_keys('mosfet')
- get_values(nodes: List | str, operations: List | str, range_start_stop: List[float | str] | None = None) Dict
Provide the applicable mean, rms, THD, ripple, Max, Min operations on the selected field that is being provided as a node to the scope.
- Parameters:
nodes (name of the signal that is provided as node to the scope block) – node names located on the scopes
operations (List or str) – Mean/RMS/THD/Ripple/Max/Min/Shape operations on the selected signals. Multiple operations can be provided as a list
range_start_stop ([float, str] or [float, float]) – the range of the data that needs to be considered for applying the mentioned operations (ex: [10e-3, start] considers data from 0 to 10e-3)
- Returns:
returns operation specific dict data (ex: operations = [mean, rms] expected returns: return_dict = {‘mean’: {‘signal_1’: mean_signal_1, ‘signal_2’: mean_signal_2}, ‘rms’: {‘signal_1’: rms_signal_1, ‘signal_2’: rms_signal_2}}
- Return type:
Dict
- open_file() None
Open the file that is provided as attribute value to the class object.
Gecko window loaded with the provided .ipes file
- run_simulation(timestep: float | None = None, simtime: float | None = None, timestep_pre: float | None = None, simtime_pre: float | None = None, save_file: bool = False) None
Run the simulation upon execution with the default time step and simulation time.
Note that file should have been opened for running the simulation.
- Parameters:
timestep (float) – the dt time step of each simulation
simtime (float) – simulation time, not including the optional pre simulation time
timestep_pre (float) – the dt time step of the pre simulation
simtime_pre (float) – simulation time of the pre simulation
save_file (bool) – True to save the file
- save_file(filename: str) None
Save the current .ipes file.
- Parameters:
filename (str) – name of the file that needs to be saved (include the path for saving into different directory)
Opened .ipes file saved under the provided name and directory.
- set_component_values(component_name: str, component_dict: Dict) None
Set the values for the configurable parameters of selected component other than switches.
- Parameters:
component_name – name of the selected component that needs to be configured
component_dict – the key value pairs that need to be set on the selected component
- Raises:
KeyError – if invalid keys are provided as the component parameters
- Example:
>>> import pygeckocircuits2 as pgc >>> gecko = pgc.GeckoSimulation('path/to/simfile.ipes') >>> gecko.set_component_values('L.1', {'iL(0)': 100})
!NOTE!: all designators and indizees must be chosen with capital letters in the .ipes file
- set_global_parameters(params_dict: Dict, save_file: bool = False) None
Set the values for the declared and defined global parameters specific to the opened .ipes file.
Note: parameters can have ‘$’ at the beginning or even not
- Parameters:
params_dict (Dict) – the name of the global parameters that are in use (works with and without ‘$’)
save_file (bool) – set to true if the current file with modified global parameters need to be saved. Default: False
- Example:
>>> import pygeckocircuits2 as pgc >>> gecko_instance = pgc.GeckoSimulation('path/to/simfile.ipes') >>> gecko_instance.set_global_parameters({'V_in': 60})
- set_loss_file(component_names: str | List, loss_file_path: str) None
Set the total loss file to the selected switch. Location of the SCL files is required for loading them into the switches.
- Parameters:
component_names (str or list) – name of the selected switch (ex: IGBT.1, MOSFET.1 etc.)
loss_file_path (str (r’C:/*/*.scl)) – the path of the .SCL file that needs to be loaded
- set_sim_time(simtime: float, timestep: float | None = None, simtime_pre: float | None = None, timestep_pre: float | None = None) None
Set the simulation time and the timestep [optional].
- Parameters:
simtime (float) – simulation time
timestep (float) – simulation time step
timestep_pre (float) – the dt time step of the pre simulation
simtime_pre (float) – simulation time of the pre simulation
- set_switch_values(sw_type: str, component_name: str, switch_key_value_dict: dict) None
Set the configuration parameters of the selected switch type. Only switch types of mosfet/igbt/diode are allowed.
- Parameters:
sw_type (str) – switch type can be either mosfet/igbt/diode
component_name (str) – name of the selected switch (ex: IGBT.1, MOSFET.1 etc.)
switch_key_value_dict (Dict) – the configuration parameter names and their values related to selected switch type that needs to be set